3 Plots In One Figure Python
- 3 Plots In One Figure Python Function
- 3 Plots In One Figure Python Morphs
- 3 Plots In One Figure Python Ide
- Python Figure Plot
- 3 Plots In One Figure Python Tutorial

Plt.subplots: The Whole Grid in One Go¶ The approach just described can become quite tedious when creating a large grid of subplots, especially if you'd like to hide the x- and y-axis labels on the inner plots. For this purpose, plt.subplots is the easier tool to use (note the s at the end of subplots). Rather than creating a single subplot. This is Scatter 3D plots with python and matplotlib. 3D Surface plots. Like line and scatter plots we can also plot surface graphs. All we have to use is plotsurface. By default it will be colored in shades of a solid color, but it also supports color mapping by supplying the cmap argument. Python functions for figures and analysis. This document is a work in progress and contains useful python functions I have discovered or written to aid in image analysis of microscopy data and making figures for presentations. Most of my work is done in python or R and I use Emacs Org-mode for literate programming. My goal for writing. Jan 18, 2021 In this example, we used the parametric equation of the circle to plot the figure using matplotlib. For this example, we took the radius of the circle as 0.4 and set the aspect ratio as 1. Method 3: Scatter Plot to plot a circle: A scatter plot is a graphical representation that makes use of dots to represent values of the two numeric values. The same as the above answer can be done with a figure object like so: import matplotlib.pyplot as plt import matplotlib.gridspec as gridspec # Create 2x2 sub plots gs = gridspec.GridSpec(2, 2) fig = plt.figure ax1 = fig.addsubplot(gs0, 0) # row 0, col 0 ax1.plot(0,1) ax2 = fig.addsubplot(gs0, 1) # row 0, col 1 ax2.plot(0,1) ax3 = fig.addsubplot(gs1,:) # row 1, span all columns.
Learning Objectives:
- To be able to produce figures with multiple plot frames, with distinct axes labels and error bars.
- To describe the interactive nature of programming to produce plots
Keywords: figure, axes, frame
In this section, we will discuss, in more detail, how Python produces plots and how we can produce multiple plots within a single programme, within the same figure.
3.3.1 Sizing and positioning plot frames
When a plot is drawn, it is drawn inside an abstract grid with its own coordinates, known as a plot frame . By default, Python chooses the size and positioning of the frame in which a plot is subsequently drawn. In particular, the bottom-left of a plot frame is taken to lie at the origin of this abstract grid, and the height and width of the plot frame are chosen to be something convenient, by default!
You can specify the size and shape of a plot frame by using the function add_axes()
. The arguments of add_axes()
are the x and y coordinates of the bottom-left of the frame, and the width and height of the frame. In particular, if the x and y coordinates of the bottom-left of the plot frame are x
and y
respectively, and the width and height of the plot frame are w
and h
respectively, the syntax to add this frame to a figure is:
plt.figure(1).add_axes((x, y, w, h))
Notice that the arguments of add_axes()
are entries enclosed in curcly brackets ( )
, instead of square brackets [ ]
as for a list. The former object is a new kind of object known as a tuple: essentially a set of entries, seperated by commas, but enclosed in curly brackets ( )
. The following example defines a tuple
For our purposes, you don’t need to know anything else about tuples, just that, in this case, they are passed to the function add_axes()
.
Let’s see how this works by adapting the example from 3.1.3 (a simple scatter plot). In this case we will set the x and y values of the bottom-left of the plot frame to be at the origin and we will set the width and height of the plot frame to be 1.5 and 0.5 respectively:
In this case, we obtain exactly the same plot obtained in example 3.1.3, but now the frame in which the plot is drawn has a size and position that we specified initially.
Exercise 3.3.1
1)
In the following code box, change the values of the entries of the tuple that is passed to add.axes()
in order to generate a plot with various different sizes and positions.
The first two entries of the tuple passed to add_axes()
can be positive or negative (to move the bottom-left corner of the frame up or down as required), but the last two entries, corresponding to the width and height of the plot, must be positive.
3.3.2 Adding and sizing/positioning a new plot frame
You can conveniently add a new plot frame to a figure, in exactly the same way as in the examples shown above, with a new position for the bottom-left of the frame and new width and height. This is how you could, for example, add a plot below (or above, or next to) an existing plot in a figure.
In the following example we will repear the example shown in 3.1.6 (Multiple plots in the same figure) and we will add a second plot frame below the first plot frame, with the same width but whose height is $0.2$ and where the top of the second frame coincices with the bottom of the first frame. We will add the same pair of plots that appear in 3.1.6 to each of the two frames:

Notice that in line-16 above, plt.figure(1)
appears again, which we recall is the syntax for creating figure 1. In this case, we aren’t creating a new figure (figure 1 already existed since line 10), but we’re adding another frame to figure 1.
3.3.3 The “active frame”
Let’s do something odd; let’s take the previous example and move the bit where we added the plot of xvalues
vs other_yvalues
(on line 19) to before the line where we created the second frame (line 16). Can you guess what will happen? Let’s see what happens:
In this case, the code adds both plots (of xvalues
vs yvalues
and xvalues
vs other_yvalues
) to the first frame. This happened because we added the second plot before we created the second plot frame. When we create a plot frame, this frame becomes the active frame : every line of code that follows it applies only to that frame , until we create another frame. In jargon we say that “the plotting environment is interactive”. This essentially means that if write code that applies something to a plot, it applies to the active frame, and to do something to a plot in another plot frame, you have to switch the active frame to the new frame. This is why the line to generate the second plot, in the second frame, had to come after the second plot frame became the active frame.
3.3.4 Removing axes ticks
Let’s consider the following example, where we have placed two identical plot frames side-by-side, using the method described in 3.2.2 . We’ve assumed we have two data sets, ranging over the same y-values, but with two sets of x-values
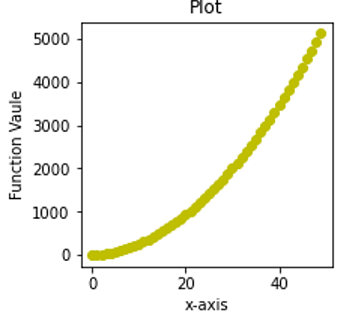
3 Plots In One Figure Python Function
An immediately unappealing feature of this figure is that the, even though these data are usefully depicted with a shared y-axis, the y-axis values are printed on the left of the right plot frame. In instances like this, you might want to just get rid of the ticks on one of the axes!
You can do this by using a combination of the functions gca()
, get_xaxis()
/get_yaxis()
and set.ticks()
.
The syntax to remove the ticks from either the x or y axis of the plot in the active frame is:

plt.gca().axes.get_xaxis().set_ticks([])
to remove the x-ticks
3 Plots In One Figure Python Morphs
plt.gca().axes.get_yaxis().set_ticks([])
to remove the y ticks
This is essentially complicated function call, but for now you can see how this works in practice and then you can simply copy and paste this line into your own code.
Going back to the previous example, we wanted to remove the ticks along the y-axis for the plot in the second plot frame. To achieve this we should add the line plt.gca().axes.get_yaxis().set_ticks([])
after the second plot frame has been created. We do this in the following example:
This looks much better!
Exercise 3.3.2
1)
Determine whether it matters if you label the axes of a plot, or change the axes limits, before or after changing the orientation of a plot.
Basic Usage – pyplot.plot
¶
Simple use of matplotlib
is straightforward:
If you run this code in the interactive Python interpreter, you should get a plot like this:
Two things to note from this plot:
pyplot.plot
assumed our single data list to be the y-values;in the absence of an x-values list, [0, 1, 2, 3] was used instead.
Note
pyplot
is commonly used abbreviated asplt
, just asnumpy
is commonlyabbreviated asnp
. The remainder of this chapter uses the abbreviatedform.Note
Enhanced interactive python interpreters such as IPython can automatesome of the plotting calls for you. For instance, you can run
%matplotlib
in IPython, after which you no longer need to runplt.show
everytime when callingplt.plot
.For simplicity,plt.show
will also be left out of the remainderof these examples.
If you pass two lists to plt.plot
you then explicitly set the x values:
Understandably, if you provide two lists their lengths must match:
To plot multiple curves simply call plt.plot
with as many x–y listpairs as needed:
Alternaltively, more plots may be added by repeatedly callingplt.plot
. The following code snippet produces the same plot as theprevious code example:
Adding information to the plot axes is straightforward to do:
3 Plots In One Figure Python Ide
Also, adding an legend is rather simple:
And adjusting axis ranges can be done by calling plt.xlim
and plt.ylim
with the lower and higher limits for the respective axes.
In addition to x and y data lists, plt.plot
can also take stringsthat define the plotting style:
Python Figure Plot
The style strings, one per x–y pair, specify color and shape: ‘rx’ standsfor red crosses, and ‘b-.’ stands for blue dash-point line. Check thedocumentationof pyplot.plot
for the list of colors and shapes.
3 Plots In One Figure Python Tutorial
Finally, plt.plot
can also, conveniently, take numpy arrays as its arguments.